Why do we need Java profilers?
In this tutorial, we will explain why we need Java profilers, and we will see visualvm
(a very popular Java profiler) in action.
Why do we need profilers?
Java profiler provides detailed information about Java applications while they are running on a Java Virtual Machine (JVM). We can use profilers to monitor Java applications. A few features of Java profilers:
- Monitor Java threads
- Monitor application memory consumption
- Display application configuration and runtime environment
- Monitor communication between Java applications and SQL database (you can find a great article on JDBC profiling here)
Why do we choose visualvm
and not some other profiler tool?
Because the visualvm
is the closest to the “official Java profiler tool”. This tool was part of JDK 6, JDK 7, and JDK 8. Only after JDK 8 (when developers of JDK decided that all tools that are not core of the JDK should be separated from JDK) visualvm
was evicted from the JDK and became a standalone tool. It was part of the JDK at some point and that is why I consider it an official Java profiler. Another advantage of the visualvm
is that it is open source and free.
How to install visualvm
?
Download visualvm
from here. Extract the zip file somewhere and run the file named visualvm.exe
which is located inside the bin
folder. You will see the home screen of the visualvm
.
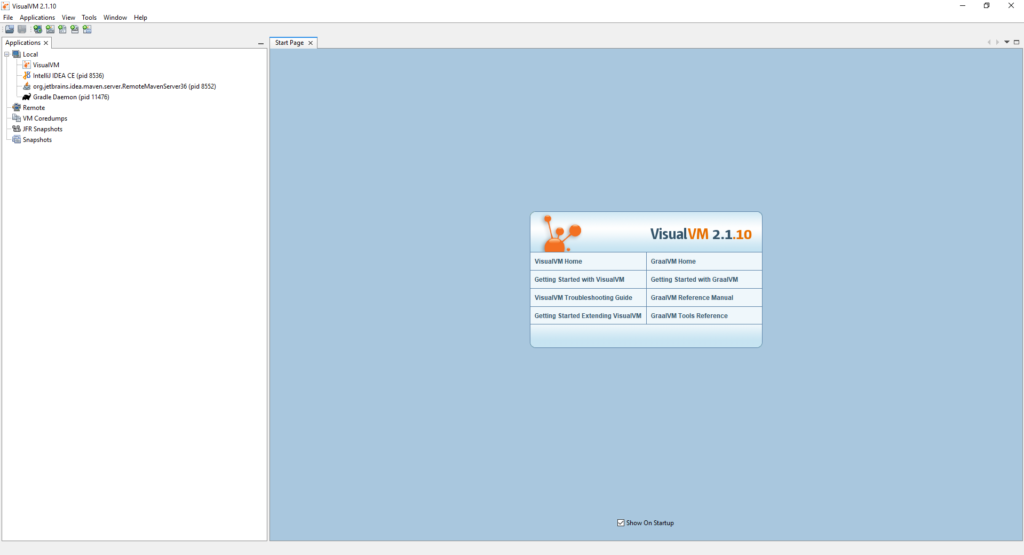
Monitoring an application
After you start visualvm
you should start your application. When you start your Java application, you will notice the name of your application in the upper left side of the visualvm
(in my case, under the Local
tab you can see the name of my application com.example.demo.DemoApplication
):
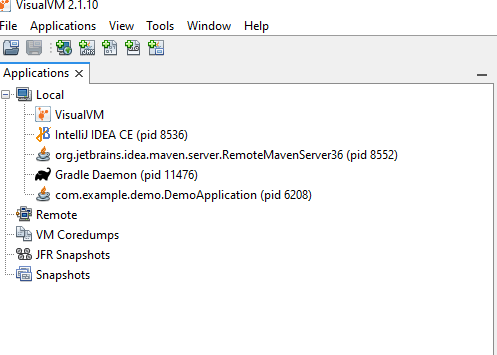
Double-click on the name of your application and we can start monitoring what’s going on in our app!
This is the starting screen of your application:
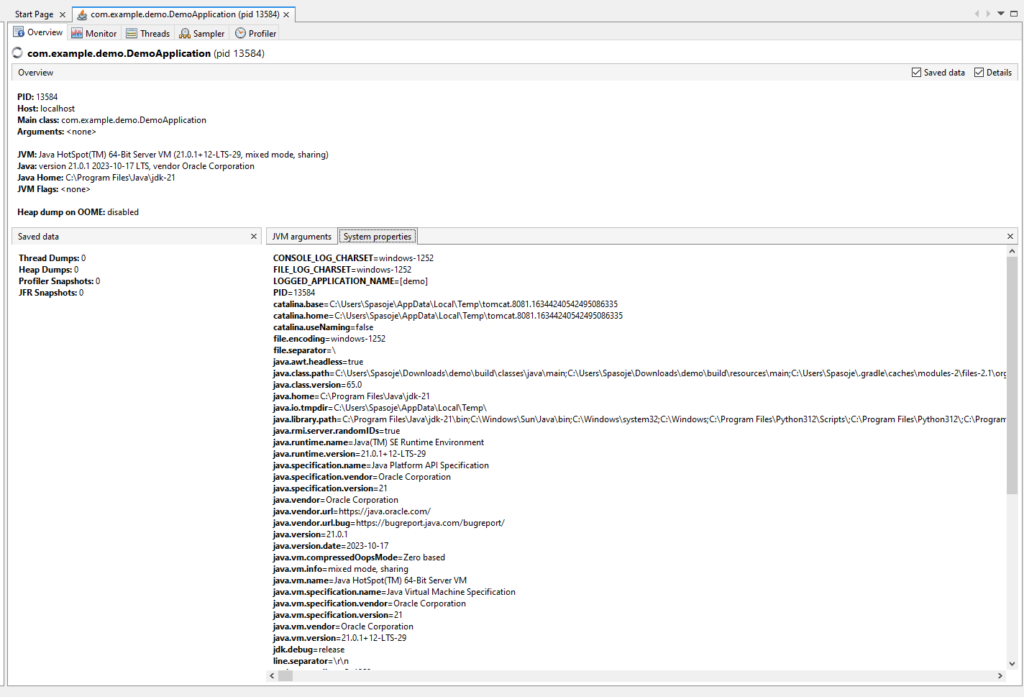
In the Overview
tab, you can see an overview of your application (Java version, Java home, JVM flags, PID, etc.). Also, you can see JVM arguments
and System properties
. This post is beginner-friendly, but it is about visualvm
so I will skip explaining basic stuff such as what is a system property, JVM argument, heap dump, thread dump, etc.
In the next Monitor tab, you can visually see four diagrams:
- CPU consumption
- Memory usage
- Data about the number of classes loaded
- Data about threads
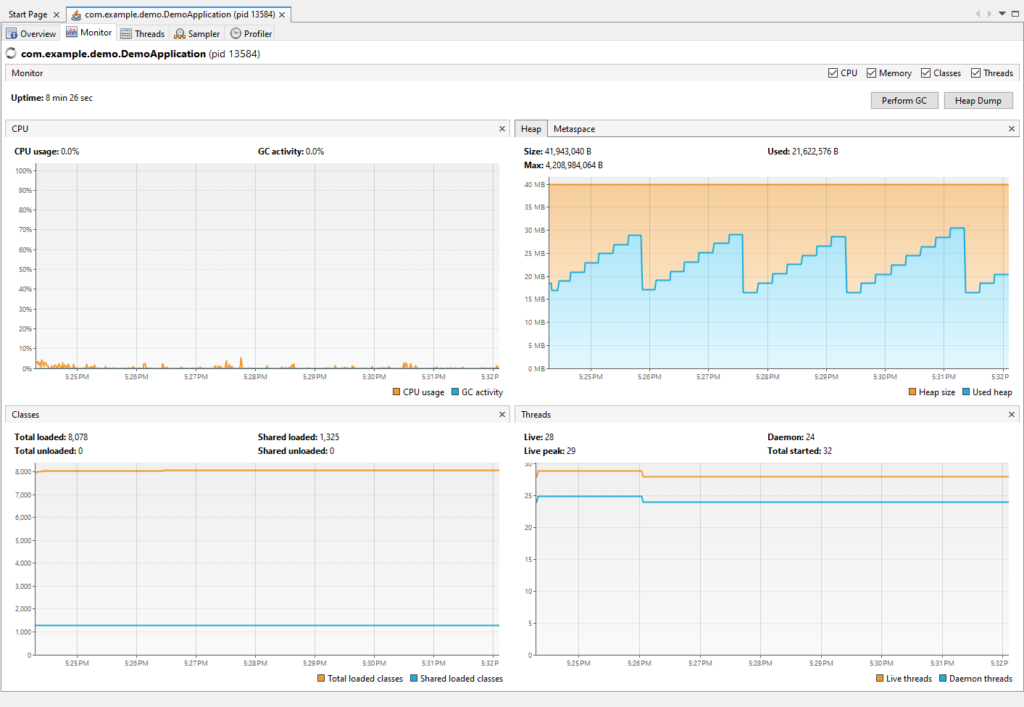
Also, in the upper right corner, there are two buttons Perform GC and Heap Dump which are self-explanatory. A heap dump is extremely helpful. If you perform a heap dump, a snapshot of your memory is created and you can analyze your memory consumption later as well. This way you can find memory problems such as memory leaks.
Analyzing heap dump
Let’s try to analyze the heap dump. For the sake of example, I wrote this code:
@Component
@Slf4j
public class MainTry implements CommandLineRunner {
List<Person> persons;
@Override
public void run(String... args) throws Exception {
Thread.sleep(10000);
PersonService personService = new PersonService();
persons = personService.createPersons();
personService.filterPersons(persons);
log.info("Inserting and filtering is done.");
}
}
public class PersonService {
public List<Person> createPersons() {
List<Person> persons = new ArrayList<>();
for(int i = 0 ; i < 50000000; i++) {
persons.add(new Person("John",(i % 10) + 1));
}
return persons;
}
public void filterPersons(List<Person> persons) {
persons.removeIf(person -> person.getAge() > 5);
}
}
Interesting note: If you make the persons
list a local variable instead of the field of the class, you will see different results in the heap dump. It doesn’t have anything to do with visualvm
. Can you figure out why the result is different?
Let’s get back to the code and explain it. It’s a simple job that waits ten seconds and then creates 50 million persons and adds them to the list. After the inserting phase, we filter only the persons older than 5.
Start your Java application and wait for the Job is done
message. Then, click on the Heap Dump button, and let’s switch to the heap dump tab. Here is what the heap dump tab looks like:
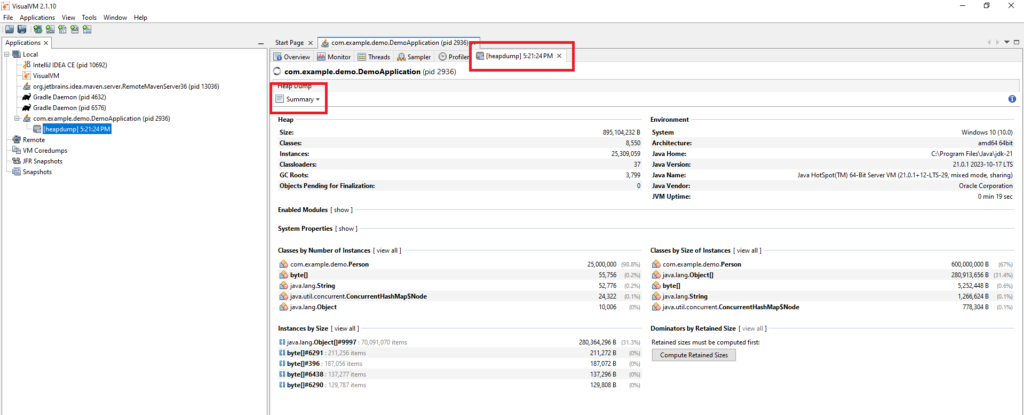
There are 25 million persons in your heap! That is because we filtered out half of the persons and Java garbage collected those person
objects. On the right side under Classes by Size of Instances
you can see that 67% of the heap size is populated with Person
objects. If you click on the Summary
(marked red on the picture) and select Objects
you can expand every object inside your heap and see the content and size of that object! Another great thing is that you can see where your object is referenced!
Analyzing single object
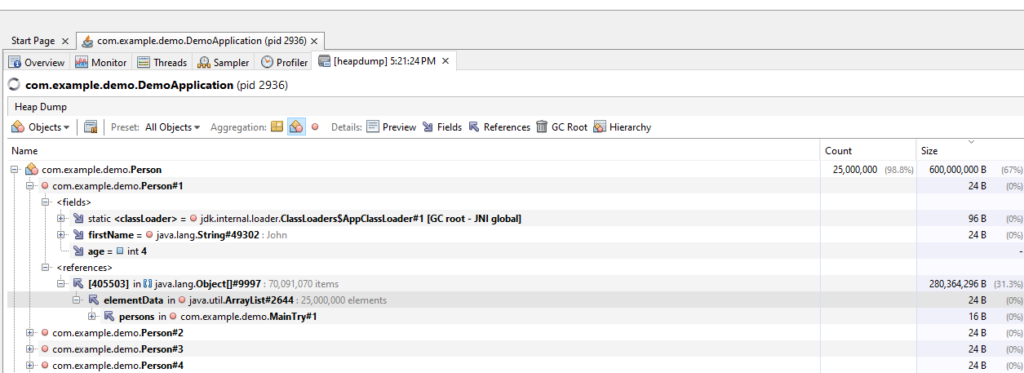
In the picture, we can see one object of class Person
. On the right side, we can see the size of an object. Also, we can see the value of the firstName
and age
fields, and under references
, we can see that object is referenced inside an ArrayList
persons
inside MainTry
class. The last thing regarding heap dump is that if you choose Threads instead of the summary(marked red on the picture) you can see the thread stack for every thread! There are plenty of other options inside the Heap Dump. Feel free to explore.
Analyzing CPU
The next question is: “Which operation takes more time(inserting or filtering)”. You can find an answer to that question inside the Sampler or Profiler tabs. Here is a great explanation what is the difference between those two tabs. In short, Profiler is more precise but slows down an application significantly. Sampler is doing samples so it does not slow down an application but is way less precise. Let’s try to compare inserting and filtering using Profiler first.
Start your application. Once you see your application in the visualvm
, click on it and go to the Profiler
tab. Click on the CPU button. It is important to start profiling(click on the CPU button) before inserting and filtering starts because only in that scenario visualvm
will profile those methods. It was different in the Heap Dump scenario because the application added objects to the memory and those objects are not going out of the memory! Here is the result of the profiling:
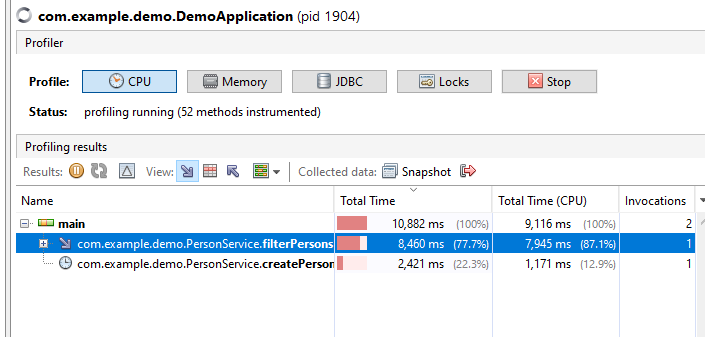
You can see that filtering is way slower compared to inserting! You can try the same measurement using Sampler. Of course, as you can guess inside the Sampler and Profiler tabs you can also analyze a memory as well. It’s just that I find it easier to create a heap dump and then analyze it.
Conclusion
And that would be it. You learned how to profile a Java application using visualvm
. You know how to see JVM parameters, system properties, how to analyze heap and thread dump(the thread dump button is inside the Threads tab, just click on it), and how to analyze memory and CPU. The last thing I want to mention is that there is a possibility to profile an application on the remote server and not only a local application. In the upper left corner instead of the local go to the Remote click Add Remote Host and just app a port and the IP of the remote app and everything will work the same!